Implementing Rate Limiting in Next.js API Routes with Upstash
Nico
2 min read
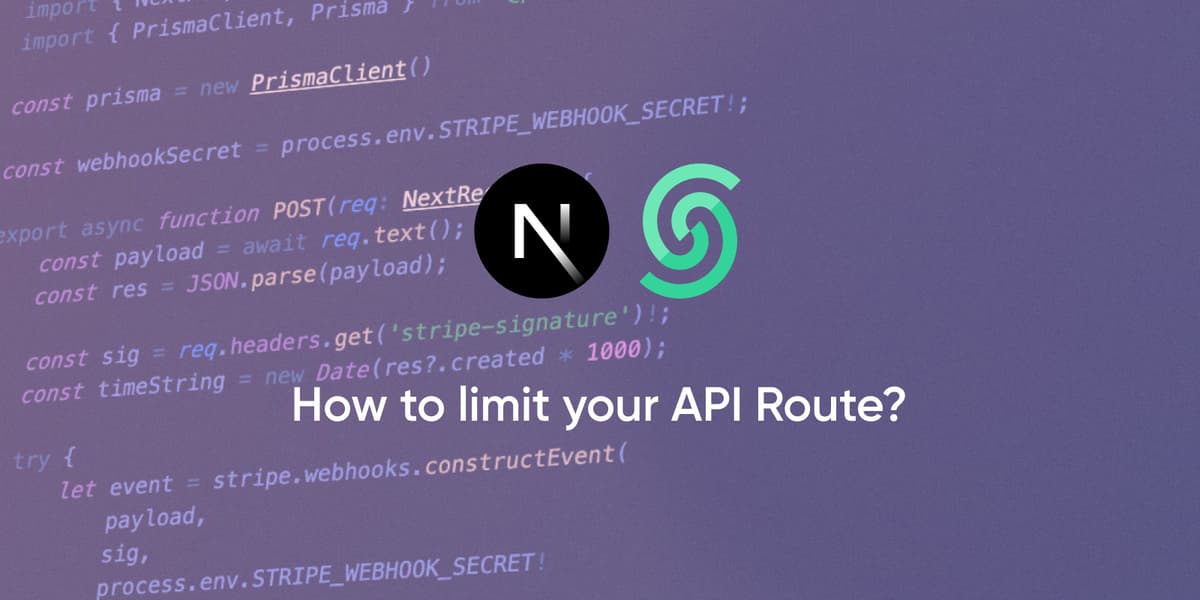
Rate limiting is a crucial aspect of API development that helps protect your services from abuse and ensures fair usage. In this quick guide, I'll show you how to implement rate limiting in Next.js API routes using @upstash/ratelimit and Vercel KV.
How It Works
- We create a rate limiter instance using @upstash/ratelimit with Vercel KV as the Redis provider.
- The fixedWindow(5, '30s') configuration allows 5 requests per 30-second window.
- For each request, we check the rate limit using the client's IP address.
- If the rate limit is exceeded, we return a 429 status code.
- Otherwise, the request proceeds normally.
Prerequisites
Before we start, make sure you have the following dependencies installed:
npm install @vercel/kv @upstash/ratelimit
Setting Up Rate Limiting
Here's a practical example of how to implement rate limiting in your Next.js API route:
import { kv } from '@vercel/kv'; import { huggingface } from '@/lib/ai/huggingface'; import { Ratelimit } from '@upstash/ratelimit'; import { NextRequest, NextResponse } from 'next/server'; // Set maximum duration for streaming responses export const maxDuration = 30; // Initialize rate limiter const ratelimit = new Ratelimit({ redis: kv, limiter: Ratelimit.fixedWindow(5, '30s'), }); export async function POST(req: NextRequest) { // Apply rate limiting based on IP address const { success, remaining } = await ratelimit.limit(req.ip ?? 'ip'); // Return 429 status if rate limit is exceeded if (!success) return new Response('Rate limit exceeded', { status: 429 }); const { prompt } = await req.json(); const output = hf.textGeneration({ model: 'meta-llama/Llama-3.2-1B', inputs: prompt, parameters: { max_new_tokens: 50, temperature: 0.7, }, }); return NextResponse.json(output); }